Inputs
An input is some knowledge about the game world that is used to calculate the score of a consideration. For example:
- My health
- Enemy’s health
- Distance to the enemy
Creating Inputs¶
There are two ways to create a new input:
-
Define a new class that inherits from
Input<TValue>
and override theOnGetRawInput
funnction. For example:
-
Define a new class that inherits from
InputFromSource<TValue>
and override theOnGetRawInput
function.
To add the input to the intelligence asset, go to the Input Tab, select the input type, give it a name, and then click the Create button:
To attach an input to an input normalization, select the input normalization in the Input Normalization Tab, and then choose the input’s name from the dropdown menu:
Note
Note: Only inputs with the same value type as the input normalization can be attached to it.
Tip
You can adjust the input values in the Intelligence Tab to observe how these changes affect the statuses of considerations and decisions. For further details, check Status Preview.
Supported Value Types¶
Currently, only the supported value types can be adjusted using the Utility Intelligence Editor to preview which decision is chosen with the Status Preview feature.
Therefore, you should use these types to enable the Status Preview feature. However, you can still use other types if you don’t need this feature. For unsupported types, you can only modify the input values by overriding OnGetRawInput()
function.
Adding Parameter Fields¶
There are many cases when you need to add parameters to an input to customize its return value. To achieve this, you need to declare these parameters as public fields in your inputs. Here are some examples of how to do this:
public abstract class InputFromSource<T> : Input<T>
{
public InputSource InputSource;
protected UtilityEntity GetInputSource(in InputContext context)
{
if (InputSource == InputSource.Self)
return Agent;
if (InputSource == InputSource.Target)
return context.Target;
return null;
}
}
public abstract class BasicInput<T> : Input<T>
{
public VariableReference<T> InputValue;
protected override T OnGetRawInput(in InputContext context)
{
return InputValue.Value;
}
}
Supported Field Types¶
Currently, only the supported field types can be serialized to JSON and adjusted using the Utility Intelligence Editor. Therefore, you should use these types when declaring parameter fields for your inputs.
Built-in Inputs¶
Currently, Utility Intelligence provides these buit-in inputs:
- BasicInputFloatInt
- BasicInputBool
- BasicInputFloat
- BasicInputDouble
- BasicInputLong
- BasicInputVector2
- BasicInputVector3
- BasicInputVector2Int
- BasicInputVector3Int
- Returns the value from its InputValue field, which can reference a variable in the Blackboard.
- DistanceToTargetInput: Returns the distance from the current agent to the target.
- CooldownElapsedTimeInput: Returns the elapsed time since the cooldown started.
- RaycastToTargetInput: Returns true if the raycast hits the target; otherwise, returns false.
If you like Utility Intelligence, please consider supporting it by leaving a 5-star review on the Asset Store.
Your positive feedback motivates me to keep improving and delivering more updates for this framework.
Thank you so much for your support. I love you all! 🥰
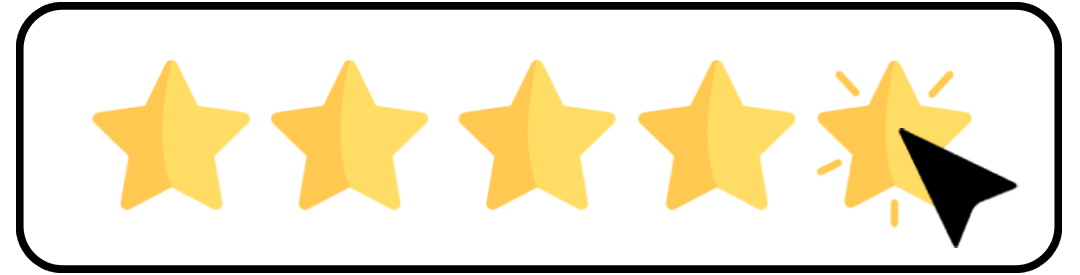
Created : September 16, 2024