Blackboard
Blackboard is used to share information between multiple components in an Agent.
- It can be access from many places, such as Inputs, Input Normalizations, Target Filters, Actions.
- It contains a list of variables and you can Read/Write to these variables for any purpose.
Creating Variables¶
To create a new variable, define a new class that inherits from Variable<TValue>
. For example:
public class FloatVariable : Variable<float>
{
public static implicit operator FloatVariable(float value)
{
return new FloatVariable { Value = value };
}
}
To add the variable to the intelligence asset, go to the Blackboard Tab, select the variable type, give it a name, and then click the Create button:
Supported Value Types¶
Currently, only the supported value types can be serialized to JSON and adjusted using the Intelligence Editor.
Therefore, you should use these types for your Blackboard Variables. However, you can still use other types if you don’t need to serialize them to JSON. For unsupported types, you need to add them to the Blackboard at runtime like this:
public class PatrolWaypoints : MonoBehaviour
{
public List<Transform> Waypoints;
private void Start()
{ Character character = GetComponent<Character>();
var blackboard = character.Entity.Intelligence.Blackboard;
var waypointsVariable = blackboard.GetVariable<TransformListVariable>(BlackboardVariableNames.Waypoints);
waypointsVariable.Value = Waypoints;
}}
Referencing Variables¶
To reference the variable from an action task, declare a public field of type VariableReference<TValue>
in the action task’s class. For example:
[Category("NavMeshAgent")]
public class MoveToTarget : NavMeshActionTask
{
public VariableReference<float> Speed = 5;
protected override void OnStart()
{
navMeshAgent.speed = Speed;
MoveToTarget();
}
protected override UpdateStatus OnUpdate(float deltaTime)
{
if (HasArrived())
return UpdateStatus.Success;
return UpdateStatus.Running;
}
protected override void OnEnd()
{
StopMove();
}
}
Then select the action task in the Decision Tab and choose the variable’s name from this dropdown menu:
Built-in Variables¶
Currently, we provides these built-in variables:
- Float
- Double
- Int
- Long
- Bool
- String
- Vector2
- Vector2Int
- Vector3
- Vector3Int
- Color
- GameObject
- GameObjectList
- Transform
- TransformList
- Animator
- NavMeshAgent
- ScriptableObject
- ScriptableObjectList
If you like Utility Intelligence, please consider supporting it by leaving a 5-star review on the Asset Store.
Your positive feedback motivates me to keep improving and delivering more updates for this framework.
Thank you so much for your support. I love you all! 🥰
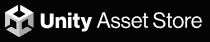
Created : September 1, 2024